일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- Q 클래스
- @IdClass
- 스프링 부트 기능
- queryDSL
- 빈생성안됨
- 복합키
- 인텔리제이
- 1차캐시
- Error creating bean with name
- uncheck Exception
- jwt메서드
- json
- jpa회원가입
- spring서버
- Spring Spring boot 차이
- 최종 프로젝트
- JPA주의사항
- ERD 작성
- git
- json gson 차이
- 스프링 부트 공식 문서
- JoinColumn
- jpa에러
- Filter
- github
- 스프링부트오류
- REST란
- JPA
- REST API 규칙
- Unsatisfied dependency
- Today
- Total
Everyday Dev System
MySelectShop - Version 1.0 본문
github 주소 :
https://github.com/Chaeyounglim/myselectshop
GitHub - Chaeyounglim/myselectshop: It's use Naver API.
It's use Naver API. Contribute to Chaeyounglim/myselectshop development by creating an account on GitHub.
github.com
초기 프로젝트 생성 및 설정 :
2023.06.22 - [내배캠 주요 학습/Spring 심화] - My Select Shop 프로젝트 초기 설정
My Select Shop 프로젝트 초기 설정
초기 프로젝트 설정 더보기 0. 프로젝트 생성 1. build.gradle에서 security 의존성 주석처리. plugins { id 'java' id 'org.springframework.boot' version '3.1.0' id 'io.spring.dependency-management' version '1.1.0' } group = 'com.sparta'
cdev.tistory.com
추가 기능:
- CRUD
- 회원가입
- 로그인
- 회원별 상품 조회
- 상품 조회 페이징 및 정렬
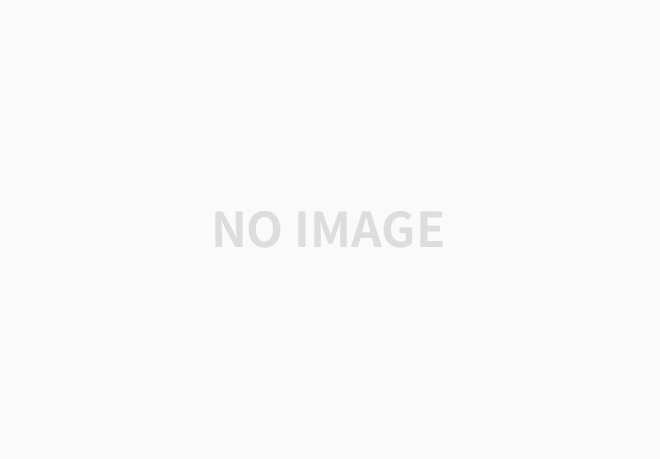
스케줄링을 활용하기 위한 방법
application 클래스에 기재 → @EnableScheduling
@EnableScheduling
@EnableJpaAuditing
@SpringBootApplication
public class MyselectshopApplication {
public static void main(String[] args) {
SpringApplication.run(MyselectshopApplication.class, args);
}
}
스케줄링 클래스 내에 아래 기재
// 초, 분, 시, 일, 월, 주 순서
@Scheduled(cron = "0 0 1 * * *") // 매일 새벽 1시
public void updatePrice() throws InterruptedException {
}
cron 이란? 특정한 시각에 자동으로 특정한 작업을 수행하게 해주고자 할 때 사용
참조 링크 :
https://docs.spring.io/spring-framework/docs/current/javadoc-api/org/springframework/scheduling/support/CronExpression.html
Product 를 조회할 때마다 User의 정보를 가져올 필요는 없다.
@ManyToOne 어노테이션의 default fetch는 EAGER(항상 조회)이다.
그러므로, 필요할 때만 조회하기 위해 fetch를 LAZY로 변경해주어야 한다.
여기서 주의할 점은 fetch가 LAZY로 되어 있을 경우에는 Transaction을 반드시 걸어줘야 함.
그 이유는, 아래와 같다.
지연 로딩이 된 Entity 정보를 조회하려고 할 때에는 반드시 영속성 컨텍스트가 존재해야 한다.
영속성 컨텍스트가 존재해야 된다는 말의 의미는 Transactional이 적용되어 있어야 한다는 의미이다.
그러므로, 아래와 같이 코드 작성 필요
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name="user_id", nullable = false)
private User user;
product.java
package com.sparta.myselectshop.entity;
import com.sparta.myselectshop.dto.ProductMypriceRequestDto;
import com.sparta.myselectshop.dto.ProductRequestDto;
import com.sparta.myselectshop.naver.dto.ItemDto;
import jakarta.persistence.*;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
@Entity // JPA가 관리할 수 있는 Entity 클래스 지정
@Getter
@Setter
@Table(name = "product") // 매핑할 테이블의 이름을 지정
@NoArgsConstructor
public class Product extends Timestamped {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String title;
@Column(nullable = false)
private String image;
@Column(nullable = false)
private String link;
@Column(nullable = false)
private int lprice;
@Column(nullable = false)
private int myprice;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name="user_id", nullable = false)
private User user;
public Product(ProductRequestDto requestDto) {
this.title = requestDto.getTitle();
this.image = requestDto.getImage();
this.link = requestDto.getLink();
this.lprice = requestDto.getLprice();
}
public void update(ProductMypriceRequestDto requestDto) {
this.myprice = requestDto.getMyprice();
}
public void updateByItemDto(ItemDto itemDto) {
this.lprice = itemDto.getLprice();
}
}
'내배캠 주요 학습 > Spring 숙련' 카테고리의 다른 글
myBlog - Version 2.1 (0) | 2023.06.26 |
---|---|
MySelectShop - Version.1.1 - 페이징 및 정렬 기능 (0) | 2023.06.23 |
My Select Shop 프로젝트 초기 설정 (0) | 2023.06.22 |
N : M 양방향 관계에서 중간 테이블 직접 생성 (1) | 2023.06.21 |
@ManyToMany 양방향 관계 이해하기 (0) | 2023.06.21 |